Lets create index.html file which will include the basic html code.
Now create a div with class menu and a header tag with class logo and three span tags to make the humburger menu (three lines) which I will animate on toggle and turn into a cross. Middle line (span tag) will fade out on click on the menu.
index.html includes style.css and script.js which has all the styles and javascript code required to make the humburger menu
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Humburger Menu</title>
</head>
<body>
<div class="menu">
<header>
<div class="logo">
<span></span>
<span></span>
<span></span>
</div>
</header>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/">About Us</a></li>
<li><a href="/">Contact Us</a></li>
</ul>
</nav>
</div>
<main>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Fermentum posuere urna nec tincidunt praesent semper feugiat. Sed elementum tempus egestas sed. Orci ac auctor augue mauris augue neque gravida. Adipiscing enim eu turpis egestas. Imperdiet massa tincidunt nunc pulvinar sapien. Massa id neque aliquam vestibulum. Eget est lorem ipsum dolor sit. Ultrices eros in cursus turpis massa tincidunt dui ut. Nulla pharetra diam sit amet nisl suscipit adipiscing bibendum est. Odio morbi quis commodo odio. Placerat vestibulum lectus mauris ultrices eros. Eu augue ut lectus arcu. Feugiat scelerisque varius morbi enim nunc faucibus a pellentesque. Nunc mi ipsum faucibus vitae.
</p>
</main>
<script src="script.js"></script>
</body>
</html>
style.css
body {
margin: 0;
padding: 0;
}
li, ul {
padding: 0;
list-style: none;
}
main {
padding: 100px;
}
.menu {
position: fixed;
width: 100%;
height: 100%;
}
header {
background: #444;
display: flex;
align-items: center;
justify-content: space-between;
padding: 20px;
box-sizing: border-box;
}
nav {
background: #333;
z-index: 0;
top: 0;
padding: 20px;
padding-top: 80px;
height: 100%;
box-sizing: border-box;
width: 200px;
left: -200px;
position: absolute;
}
nav a {
color: #fff;
display: block;
margin-bottom: 6px;
font-size: 1.2em;
}
.logo {
z-index: 1;
display: relative;
font-size: 1.2em;
cursor: pointer;
}
.logo span {
display: block;
width: 30px;
height: 2px;
background: #eee;
margin-bottom: 5px;
}
nav.open {
left: 0;
}
.logo.open span:nth-child(1) {
transform: rotate(45deg) translateY(10px);
}
.logo.open span:nth-child(2) {
opacity: 0;
width: 0;
}
.logo.open span:nth-child(3) {
transform: rotate(-45deg) translateY(-10px);
}
nav {
transition: left ease-in 0.3s;
}
.logo span {
transition: all ease-in 0.3s;
}
script.js
const humburger = document.querySelector('.logo');
const navbar = document.querySelector('nav');
humburger.addEventListener('click', (e) => {
navbar.classList.toggle('open')
humburger.classList.toggle('open')
});
Final result will look like as shown in below screenshots:
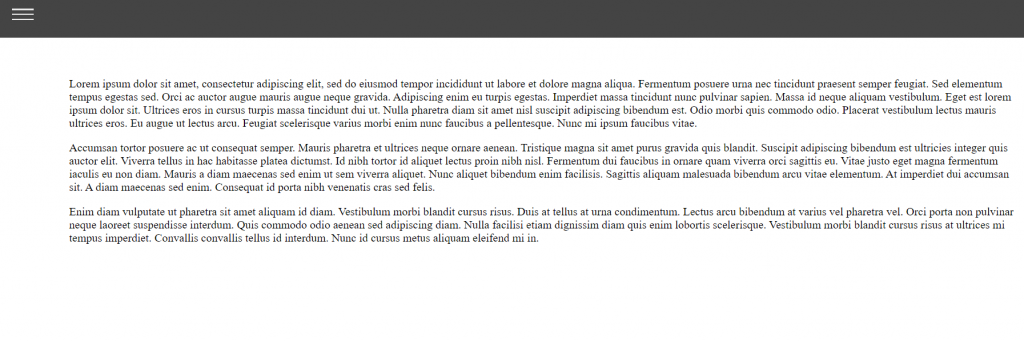
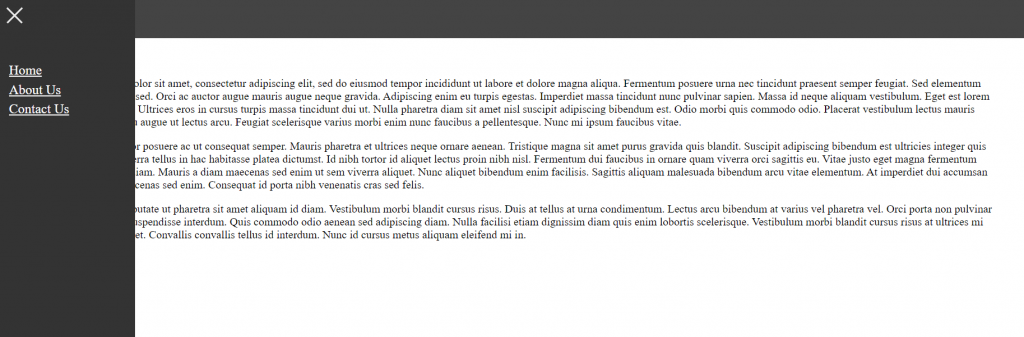
Full code can be found at my github repo.