Create below three files in a folder
index.html
app.js
style.css
We will be using Vue js CDN version 3.0.5<script src=”https://unpkg.com/[email protected]″></script>
First we will create index.html with below code. In this code id=”app” will display the output based on the Vue components.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width,initial-scale=1.0" />
<title>Generate Random User</title>
</head>
<body>
<div id="app"></div>
<script src="https://unpkg.com/[email protected]"></script>
<script src="app.js"></script>
</body>
</html>
Now create vue app using below code and print “Hello World!!”
const app = Vue.createApp({
template: '<h1> Hello World!!! </h1>',
});
app.mount('#app');
Now you can run the code on live server and see index.html will display Hello World!! on the page.
Now lets add data function to return object from Vue app and you will get Hello John output on the index.html
const app = Vue.createApp({
template: '<h1> Hello {{ firstName }} <h1>',
data () {
return {
firstName: "John"
}
}
});
app.mount('#app');
Now add the template part to the index.html code inside the div tag where id=”app”
Remove the template part and add it to the index.html
app.js will be as below
const app = Vue.createApp({
template: '<h1> Hello {{ firstName }} <h1>',
data () {
return {
firstName: "John"
}
}
});
app.mount('#app');
index.html – you will see it still displays Hello John
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width,initial-scale=1.0" />
<title>Generate Random User</title>
</head>
<body>
<div id="app">
<h1>Hello {{ firstName }} </h1>
</div>
<script src="https://unpkg.com/[email protected]"></script>
<script src="app.js"></script>
</body>
</html>
Now lets add more details to the user data in app.js
const app = Vue.createApp({
template: '<h1> Hello {{ firstName }} <h1>',
data () {
return {
firstName: "John",
lastName: "Doe",
email: "[email protected]",
gender: "Male",
picture: 'https://randomuser.me/api/portraits/men/20.jpg'
}
}
});
app.mount('#app');
And update these details in index.html and also add class gender in the html code using v-bind (:)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width,initial-scale=1.0" />
<title>Generate Random User</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="app">
<img :class="gender"
:src="picture"
:alt="` ${ firstName } ${ lastName }`">
<h1> {{ firstName }} {{ lastName }} </h1>
<h3>Email: {{ email }} </h3>
<button :class="gender" >Generate Random User </button>
</div>
<script src="https://unpkg.com/[email protected]"></script>
<script src="app.js"></script>
</body>
</html>
Now lets fetch user details from the api call dynamically
Add getUser() method to the app.js and on button click event to the index.html
Final code will look like below
app.js
const app = Vue.createApp({
data () {
return {
firstName: "John",
lastName: "Doe",
email: "[email protected]",
gender: "male",
picture: 'https://randomuser.me/api/portraits/men/20.jpg'
}
},
methods: {
async getUser() {
const res = await fetch("https://randomuser.me/api");
const { results } = await res.json()
console.log(results)
this.firstName = results[0].name.first
this.lastName = results[0].name.last
this.email = results[0].email
this.gender = results[0].gender
this.picture = results[0].picture.large
},
},
});
app.mount('#app');
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width,initial-scale=1.0" />
<title>Generate Random User</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="app">
<img :class="gender"
:src="picture"
:alt="` ${ firstName } ${ lastName }`">
<h1> {{ firstName }} {{ lastName }} </h1>
<h3>Email: {{ email }} </h3>
<button v-on:click="getUser()" :class="gender" >Generate Random User </button>
</div>
<script src="https://unpkg.com/[email protected]"></script>
<script src="app.js"></script>
</body>
</html>
style.css
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
html,
body {
font-family: Arial, Helvetica, sans-serif;
}
#app {
width: 400px;
height: 100vh;
margin: auto;
text-align: center;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
h1,
h3 {
margin-bottom: 1rem;
font-weight: normal;
}
img {
border-radius: 50%;
border: 5px #333 solid;
margin-bottom: 1rem;
}
.male {
border-color: steelblue;
background-color: steelblue;
}
.female {
border-color: pink;
background-color: pink;
color: #333;
}
button {
cursor: pointer;
display: inline-block;
background: #333;
color: white;
font-size: 16px;
border: 0;
padding: 1rem 1.5rem;
border-radius: 50px;
}
button:focus {
outline: none;
}
button:hover {
transform: scale(0.98);
}
After all these updates you should be able to see Generate user as below
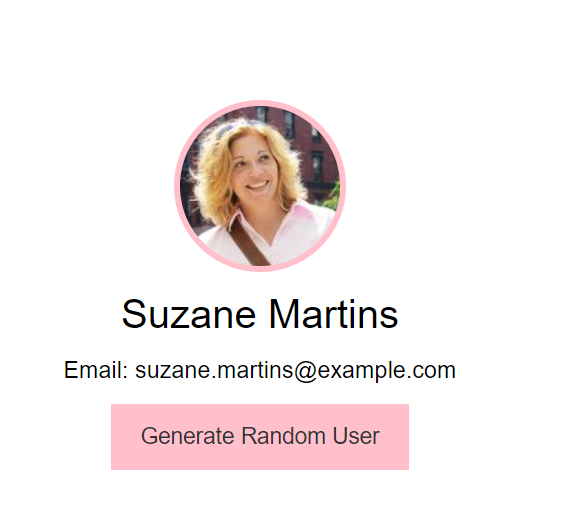
Hope this is helpful and let me know if you have any questions in the comments box 🙂
Complete demo can be viewed here Generate Random User