Google Geocoding API to display address details including latitude and longitude
- Create your own Google API key and update
YOUR_API_KEY
in the src/script.js - Use HTML, Javascript and CSS
- Include axios CDN or you can use npm install
- Inlude bootstrap CDN or use npm install
- Address search results using Google Geocodig API
Step 1 – create an index.html file which will accept unformatted user input as address and then display address results along with latitude and longitude on click on submit button.
Include Bootstrap 5 CDN to use css classes from bootstrap library to format the address output on html page.
Include axios library CDN to make axios.get request to fetch data from google geocode api.
Include script.js file which is used to create all axios get requests to fetch data and return desired output data.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Google Geocoding API to display address details</title>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
</head>
<body>
<!--The div element for the map -->
<div class="container p-5">
<h3 class="card-title">Display address details using Google Geocoding API</h3>
<form id="address-form">
<div class="form-group m-3">
<label class="form-label">Enter location details here</label>
<input type="text" id="address" class="form-control"/>
</div>
<button type="submit" class="btn btn-primary m-3">Submit</button>
</form>
<div class="m-3">
<div id="formatted-address"></div>
</div>
<div class="m-3">
<div id="address-components"></div>
</div>
<div class="m-3">
<div id="geometry"></div>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
script.js
- Get address from the form where form id is address-form
- Create a function to get the geocode
- Call getGeocode() when the button is clicked
- Create axios get request to fetch data from google geoscope api https://maps.googleapis.com/maps/api/geocode/json
- Get the formatted address response and insert it into the DOM using innerHTML
- Get the address components from response and insert into DOM using innerHTML
- Get latitude and longitude from response and insert into DOM using innerHTML
//Get address form
var addressForm = document.getElementById("address-form");
// Call getGeocode() when the button is clicked
addressForm.addEventListener('submit', getGeocode);
// Create a function to get the geocode
function getGeocode(e) {
// Prevent the form from submitting
e.preventDefault();
let location = document.getElementById("address").value;
axios.get("https://maps.googleapis.com/maps/api/geocode/json", {
params: {
address: location,
key: 'YOUR_API_KEY'
}
})
.then(function(response) {
//Get formatted address from response
var formattedAddress = response.data.results[0].formatted_address;
var formattedAddressOutput = `
<ul class="list-group">
<li class="list-group-item">${formattedAddress}</li>
</ul>
`;
//Set inner HTML of the geocode div with formatted address
document.getElementById('formatted-address').innerHTML = formattedAddressOutput;
//Get address components from response
var addressComponents = response.data.results[0].address_components;
//Create addressComponentsOutput variable to hold the output
var addressComponentsOutput = `<ul class="list-group">`;
//Loop through address components
for(var i = 0; i < addressComponents.length; i++) {
addressComponentsOutput += `<li class="list-group-item"><strong>${addressComponents[i].types[0]}</strong>: ${addressComponents[i].long_name}</li>`;
}
addressComponentsOutput += `</ul>`;
//Set inner HTML of the geocode div with formatted address
document.getElementById('address-components').innerHTML = addressComponentsOutput;
//Get latitude and longitude from response
var lat = response.data.results[0].geometry.location.lat;
var lng = response.data.results[0].geometry.location.lng;
var geometryOutput = `
<ul class="list-group">
<li class="list-group-item"><strong>Latitude</strong>: ${lat}</li>
<li class="list-group-item"><strong>Longitude</strong>: ${lng}</li>
</ul>
`;
//Set inner HTML for the latitude and longitude
document.getElementById('geometry').innerHTML = geometryOutput;
})
.catch(function(error){
console.log(error);
});
}
Here is the screenshot of address results using Geocoding API:
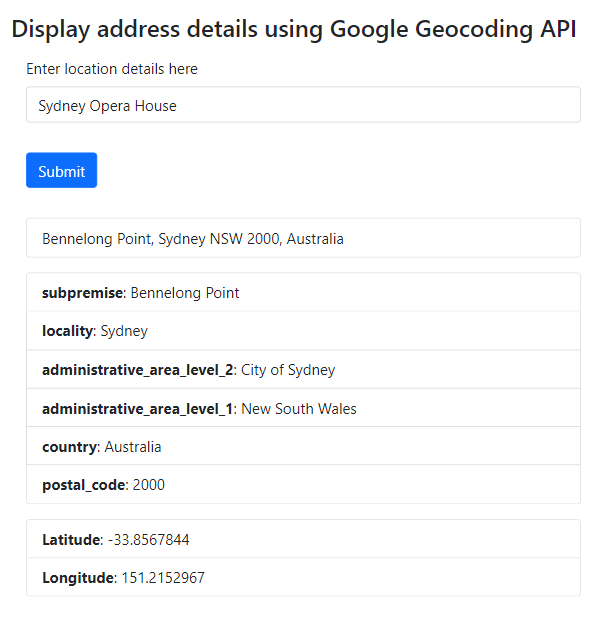
Hope this helped you in learning and developing your own application to display address results with latitude and longitude which can be saved in database. If you have any questions feel free to reach me here.
Full source code for this project can be downloaded from my Github repo here