Google Maps API to display map with different types of markers for multiple locations
First you will need to get Google Maps API key from below link:
Update this API key at YOUR_API_KEY in index.html
Step 1 – create an index.html which is used to display map in the div section where id is map.
Include style.css in the head section and script.js files just above the closing body tag.
Include Google maps api key js https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>Display maps with multiple markers using Google Maps API</title>
</head>
<body>
<h1>Display maps with different types of Markers</h1>
<!--The div element for the map -->
<div id="map"></div>
<script src="script.js"></script>
<!-- Async script executes immediately and must be after any DOM elements used in callback. -->
<script async defer
src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap"
></script>
</body>
</html>
Step 2 – Now let’s create script.js which will include all necessary code to display maps for different locations.
Define map options – zoom level and center coordinates
Create a new map object and specify the DOM element for display
Create a marker icon svg image
Create an array of locations with different iconImages i.e, png image, svg image and no image (dault icon)
Loop through locations and add markers
Create a marker and set its position
Add on click event listener to marker and open info window
script.js
function initMap() {
// Define map options - zoom level and center coordinates
var options = {
zoom: 4,
center : {lat: -27.4705, lng: 153.0260}
}
// Create a new map object and specify the DOM element for display
var map = new google.maps.Map(document.getElementById('map'), options);
// Create a marker icon svg image
const svgMarker = {
path: "M10.453 14.016l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM12 2.016q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z",
fillColor: "blue",
fillOpacity: 0.6,
strokeWeight: 0,
rotation: 0,
scale: 2,
anchor: new google.maps.Point(15, 30),
};
// Create an array of locations with different iconImages i.e, png image, svg image and no image (default icon)
var locations = [
{
coordinates: {lat: -27.4705, lng: 153.0260},
iconImage: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png",
content: '<h3>Brisbane</h3>'
},
{
coordinates: {lat: -33.8688, lng: 151.2093},
iconImage: svgMarker,
content: '<h3>Sydney</h3>'
},
{
coordinates: {lat: -37.8136, lng: 144.9631},
content: '<h3>Melbourne</h3>'
}
];
// Loop through locations and add markers
for (let i = 0; i < locations.length; i++) {
//Add marker
addMarker(locations[i]);
}
// Create a marker and set its position
function addMarker(props) {
// Create a marker and set its position along with marker title and content
var marker = new google.maps.Marker({
position: props.coordinates,
map: map
});
// Check for custom icon
if (props.iconImage) {
// Set icon image
marker.setIcon(props.iconImage);
}
// Check content
if(props.content) {
// Create an info window and set its content
var infoWindow = new google.maps.InfoWindow({
content: props.content
});
}
// Add on click event listener to marker and open info window
marker.addListener('click', function() {
infoWindow.open(map, marker);
});
}
}
Step -3 – Create a style.css file and give a height of 400px and width 100% to the map.
style.css
#map {
height: 400px;
width: 100%;
}
Here is the screenshot of map after implementing Google Maps API with above code:
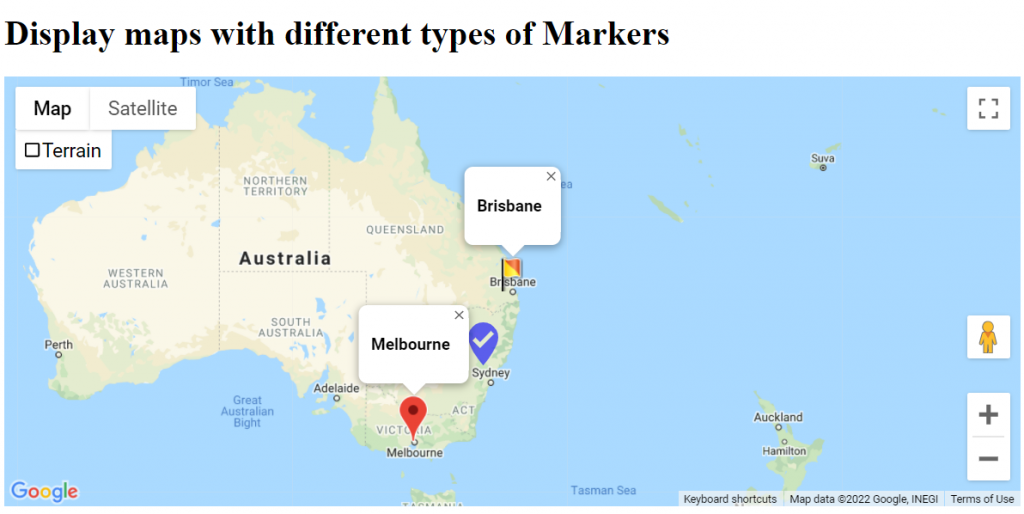
Hope this helped you in learning and developing your own application to display map locations with different markers. If you have any questions feel free to reach me here.
Full source code for this project can be downloaded from my Github repo here